Unorthodox Learning Style
W3 Schools, Free Code Academy, and YouTube
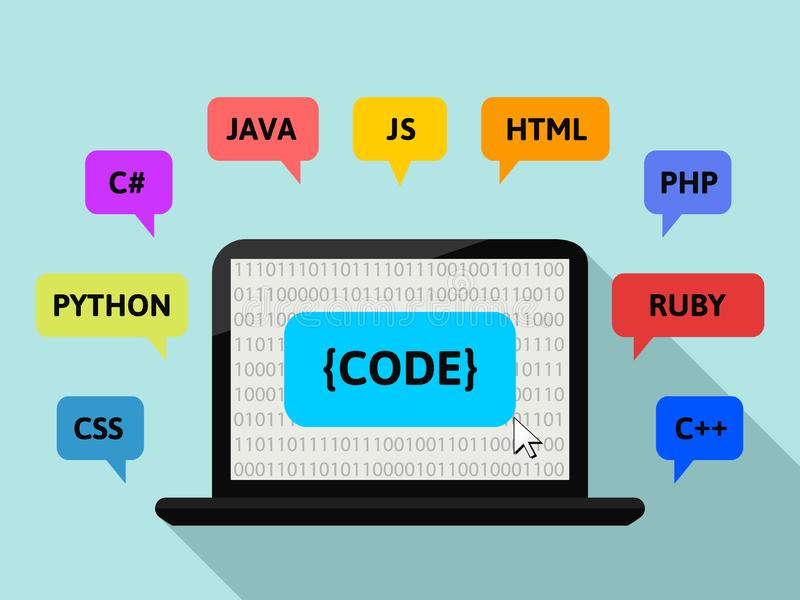
With the dawn of websites such as YouTube, GeeksForGeeks, W3Schools, and FreeCodeAcademy learning how to code on your own has never been easier. No longer are issues such as time or accessibility an issue when said resources are available on the internet to be accessed whenever you want. Which is where I first gained my introduction to the world of coding.
Initially, the first serious coding introduction I got was through W3School, where I got introduced to web development languages like HTML, CSS, JavaScript. It was here, that I got my first foothold into web developing, and was later introduced to backend developing using PHP?, SQL, and Java.
This introduction is what later factored into my decision to take AP Computer Science and take AP Computer Science A in my senior year. As of the time of writing this, I am in the second and final semester of my senior year and en route to finish my AP Computer Science pathway. Some projects I have completed including both school projects and personal projects are A basic 4-function Calculator using java (school), a Palindrome program using Java (school), a simple photo editor that lets you choose between a set number of filters in Python (personal), a tic-tac-toe game using Java (school), and this very website (personal).
Palindrome Program:
import java.util.Scanner;
public class Main
{
public static void main(String[] args) {
String original, reverse = "";
Scanner in = new Scanner(System.in);
System.out.println("Enter a sting: ");
original = in.nextLine();
int length = original.length();
for ( int i = length - 1; i >= 0; i-- )
reverse = reverse + original.charAt(i);
if (original.equals(reverse))
System.out.println("Entered string/number is a palindrome.");
else
System.out.println("Entered string/number isn't a palindrome.");
}
}
4 Function Calculator:
import java.util.Scanner;
public class Main{
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.println("Enter first number: ");
double firstNum = input.nextDouble();
System.out.println("Enter second number: ");
double secondNum = input.nextDouble();
System.out.println("Enter third number: ");
double thirdNum = input.nextDouble();
System.out.println("Enter the operation");
System.out.println("For addition + ");
System.out.println("For subtraction - ");
System.out.println("For multiplication * ");
System.out.println("For division / : ");
char oper = input.next().charAt(0);
if(oper == '+') {
System.out.println("The sum of "+firstNum+" and "+secondNum+" and "+thirdNum+" = "+(firstNum+secondNum+thirdNum));
}else if(oper == '-') {
System.out.println("The subtraction of "+firstNum+" and "+secondNum+" and "+thirdNum+" = "+(firstNum-secondNum-thirdNum));
}else if(oper == '*') {
System.out.println("The multiplication of "+firstNum+" and "+secondNum+" and "+thirdNum+" = "+(firstNum*secondNum*thirdNum));
}else if(oper == '/') {
System.out.println("The division of "+firstNum+" and "+secondNum+" and "+thirdNum+" = "+(firstNum/secondNum/thirdNum));
}else {
System.out.println("Invalid Input");
}
}
}